Consider the control flow graph shown.
Which one of the following choices correctly lists the set of live variables at the exit point of each basic block?
Consider the following ANSI C code segment:
z = x + 3 + y -> f1 + y -> f2;
for (i = 0; i < 200; i = i + 2){
if (z > i) {
P = p + x + 3;
q = q + y -> f2;
} else {
p = p + y -> f2;
q = q + x + 3;
}
}
Assume that the variable y points to a struct (allocated on the heap) containing two fields f1 and f2, and the local variables x, y, z, p, g, and i are allotted registers. Common sub-expression elimination (CSE) optimization is applied on the code. The number of addition and dereference operations (of the form y -> f1 or y -> f2 ) in the optimized code, respectively, are:
For a statement S in a program, in the context of liveness analysis, the following sets are defined:
USE(S): the set of variables used in S
IN(S): the set of variables that are live at the entry of S
OUT(S): the set of variables that are live at the exit of S
Consider a basic block that consists of two statements, S1 followed by S2.
Which one of the following statements is correct?
i. There exists a statement $${S_j}$$ that uses x
ii. There is a path from $${S_i}$$ to $${S_j}$$ in the flow graph corresponding to the program
iii. The path has no intervening assignment to x including at $${S_i}$$ and $${S_j}$$
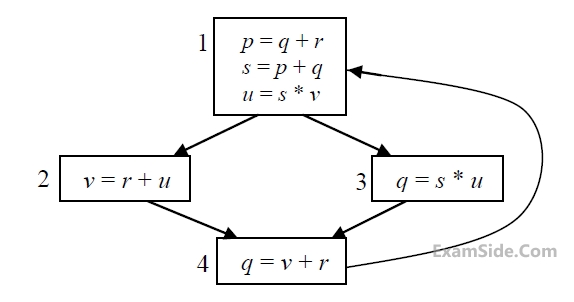
The variables which are live both at the statement in basic block 2 and at the statement in basic block 3 of the above control flow graph are