Complexity Analysis and Asymptotic Notations · Algorithms · GATE CSE
Marks 1
Consider an unordered list of $N$ distinct integers. What is the minimum number of element comparisons required to find an integer in the list that is NOT the largest in the list?
Consider the following recurrence relation :
$$T(n)=2 T(n-1)+n 2^n \text { for } n>0, T(0)=1$$
Which ONE of the following options is CORRECT?
Let $T(n)$ be the recurrence relation defined as follows:
$T(0) = 1$
$T(1) = 2$, and
$T(n) = 5T(n - 1) - 6T(n - 2)$ for $n \geq 2$
Which one of the following statements is TRUE?
Given an integer array of size $N$, we want to check if the array is sorted (in either ascending or descending order). An algorithm solves this problem by making a single pass through the array and comparing each element of the array only with its adjacent elements. The worst-case time complexity of this algorithm is
Let $$f$$ and $$g$$ be functions of natural numbers given by $$f(n)=n$$ and $$g(n)=n^2$$. Which of the following statements is/are TRUE?
Which one of the following statements is TRUE for all positive functions f(n) ?
Consider the following three functions.
f1 = 10n, f2 = nlogn, f3 = n√n
Which one of the following options arranges the functions in the increasing order of asymptotic growth rate?
T(n) = $$T\left( {{n^{1/a}}} \right) + 1$$, and T(b) = 1.
Then T(n) is
$$\eqalign{ & \,\,\,\,\,\,\,{\rm I}.\,\,\,\,\,\,\Theta \left( {{n^4}} \right) \cr & \,\,\,\,\,{\rm I}{\rm I}.\,\,\,\,\,\,\Theta \left( {{n^5}} \right) \cr & \,\,\,{\rm I}{\rm I}{\rm I}.\,\,\,\,\,\,O\left( {{n^5}} \right) \cr & \,\,\,{\rm I}V.\,\,\,\,\,\,\Omega \left( {{n^3}} \right) \cr} $$
The equality above remains correct if $$𝑋$$ is replaced by
int j, n;
j=1;
while(j <= n)
j = j * 2;
The number of comparisons made in the execution of the loop for any n > 0 is:for (i = n, j = 0; i > 0; i /= 2, j += i);
let val (j) denote the value stored in the variable j after termination of the for loop. Which one of the following is true?
I. (n + k)m = $$\Theta \,({n^m})$$ where k and m are constants
II. 2n+1 = O(2n)
III. 22n = O(22n)
Which of those claims are correct?
Marks 2
Consider the following recurrence relation:
$$T(n) = \begin{cases} \sqrt{n} T(\sqrt{n}) + n & \text{for } n \ge 1, \\ 1 & \text{for } n = 1. \end{cases}$$
Which one of the following options is CORRECT?
Consider functions Function_1 and Function_2 expressed in pseudocode as follows:
Function 1
while n > 1 do
for i = 1 to n do
x = x + 1;
end for
n = n/2;
end while
Function 2
for i = 1 to 100 ∗ n do
x = x + 1;
end for
Let $$f_1(n)$$ and $$f_2(n)$$ denote the number of times the statement "$$x=x+1$$" is executed in Function_1 and Function_2, respectively.
Which of the following statements is/are TRUE?
Consider the following recurrence :
f(1) = 1;
f(2n) = 2f(n) $$-$$ 1, for n $$\ge$$ 1;
f(2n + 1) = 2f(n) + 1, for n $$\ge$$ 1;
Then, which of the following statements is/are TRUE?
For constants a ≥ 1 and b > 1, consider the following recurrence defined on the non-negative integers :
$$T\left( n \right) = a.T\left( {\frac{n}{b}} \right) + f\left( n \right)$$
Which one of the following options is correct about the recurrence T(n)?
Consider the following recurrence relation.
$$T(n) = \left\{ {\begin{array}{*{20}{c}} {T(n/2) + T(2n/5) + 7n \ \ \ if\ n > 0}\\ {1\ \ \ \ \ \ \ if\ n = 0} \end{array}} \right.$$
Which one of the following option is correct?
There are n unsorted arrays : A1, A2, …, An. Assume that n is odd. Each of A1, A2, …, An contains n distinct elements. There are no common elements between any two arrays. The worst-case time complexity of computing the median of the medians of A1, A2, …, An is :
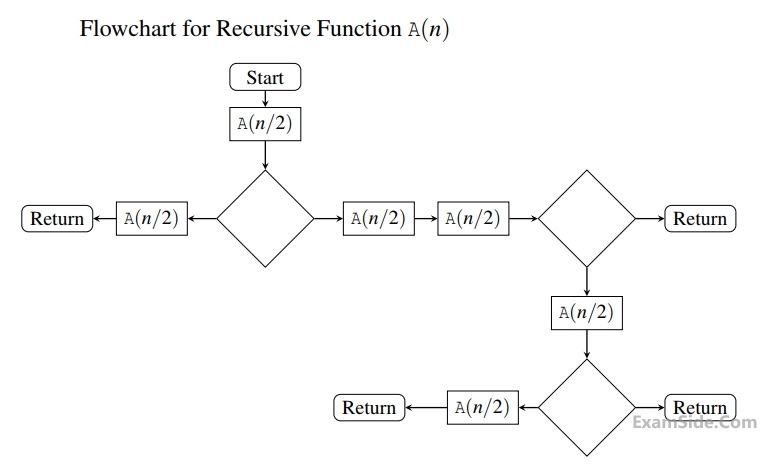
$$\eqalign{ & \,\,\,\,\,\,\,{\rm I}.\,\,\,\,\,\,\,f\left( n \right) = O\left( {g\left( n \right)} \right) \cr & \,\,\,\,\,{\rm I}{\rm I}.\,\,\,\,\,\,\,f\left( n \right) = \Omega \left( {g\left( n \right)} \right) \cr} $$
int fun1 (int n) {
int i, j, k, p, q = 0;
for (i = 1; i < n; ++i)
{
p = 0;
for (j = n; j > 1; j = j/2)
++p;
for (k = 1; k < p; k = k * 2)
++q;
}
return q;
}
Which one of the following most closely approximates the return value of the function fun1?f1 = 2n f2 = n3/2
f3(n) = $$n\,\log _2^n$$
f4 (n) = n log2n
int f1(int n){
if(n == 0 || n == 1){
return n;
}
return (2 * f1(n - 1) + 3 * f1(n - 2));
}
int f2(int n){
int i;
int X[N], Y[N], Z[N];
X[0] = Y[0] = Z[0] = 0;
X[1] = 1; Y[1] = 2; Z[1] = 3;
for(i = 2; i <= n; i++){
X[i] = Y[i - 1] + Z[i - 2];
Y[i] = 2 * X[i];
Z[i] = 3 * X[i];
}
return X[n];
}
f1(8) and f2(8) return the values int f1(int n){
if(n == 0 || n == 1){
return n;
}
return (2 * f1(n - 1) + 3 * f1(n - 2));
}
int f2(int n){
int i;
int X[N], Y[N], Z[N];
X[0] = Y[0] = Z[0] = 0;
X[1] = 1; Y[1] = 2; Z[1] = 3;
for(i = 2; i <= n; i++){
X[i] = Y[i - 1] + Z[i - 2];
Y[i] = 2 * X[i];
Z[i] = 3 * X[i];
}
return X[n];
}
The returning time of f1(n) and f2(n) areG(n) = n!
H(n) = nlogn
Which of the following statements about the asymptotic behaviour of f(n), g(n), and h(n) is true?
int gcd(n,m)
{
if (n % m == 0) return m;
n = n % m;
return gcd(m,n);
}
How many recursive calls are made by this function?int DoSomething(int n){
if(n <= 2)
return 1;
else
return (floor(sqrt(n)) + n);
}
int IsPrime(n){
int i, n;
for(i=2; i<=sqrt(n);i++){
if(n % i == 0){
printf("No prime\n"); return 0;
}
return 1;
}
}
Let T(n) denote the number of times the for loop is executed by the program on input n. Which of the following is TRUE?double foo(int n){
int i;
double sum;
if(n == 0) return 1.0;
sum = 0.0;
for (i = 0; i < n; i++){
sum += foo(i);
}
return sum;
}
Suppose we modify the above function foo() and store the values of foo(i), $$0 \le i \le n$$, as and when they are computed. With this modification, the time complexity for function foo() is significantly reduced. The space complexity of the modified function would be:double foo(int n){
int i;
double sum;
if(n == 0) return 1.0;
sum = 0.0;
for (i = 0; i < n; i++){
sum += foo(i);
}
return sum;
}
The space complexity of the above function is:10, 8, 5, 3, 2
Two new elements '1' and '7' are inserted in the heap in that order, The level order traversal of the heap after the insertion of the elements is:
T(1) = 1
T(n) = 2T(n - 1)+n, $$n \ge 2$$
Evaluates to
int recursive(int n){
if(n == 1){
return (1);
}
return (recursive(n - 1) + recursive(n - 1));
}
counter = 0;
for(i = 1; i <= n; i++){
if(A[i]==1){
counter++;
}else{
f(counter); counter = 0;
}
}
The complexity of this program fragment is(Assume m > 1, $$ \in > 0$$)
x = m;
y = 1;
while(x - y > ε){
x = (x + y) / 2;
y = m/x;
}
print(x);
If n<=2 return (1) else return (A([$$\sqrt n $$])); is best described by
1. Choose an i uniformly at random fro 1..n;
2. If A[i]=x then stop else Goto 1;
Assuming that x is present A, what is the expected number of comparisons made by the algorithm before it terminates?
$$f(n) = 3{n^{\sqrt n }}$$
$$g(n) = {2^{\sqrt n {{\log }_2}n}}$$
$$h(n) = n!$$
Which of the following is true?
$${g_1}(n) = \left\{ {\matrix{ {{n^3}\,for\,0 \le n < 10,000} \cr {{n^2}\,for\,n \ge 10,000} \cr } } \right.$$
$${g_2}(n) = \left\{ {\matrix{ {n\,for\,0 \le n \le 100} \cr {{n^3}\,for\,n > 100} \cr } } \right.$$ Which of the following is true:
T (n) = T (n - 1) + n
T (1) = 1