Code Generation and Optimization · Compiler Design · GATE CSE
Marks 1
Which ONE of the following techniques used in compiler code optimization uses live variable analysis?
I. Symbol table is accessed only during lexical analysis and syntax analysis.
II. Compilers for programming languages that support recursion necessarily need heap storage for memory allocation in the run-time environment.
III. Errors violating the condition ‘any variable must be declared before its use’ are detected during syntax analysis.
Which of the above statements is/are TRUE?
Match the followings:
Group-I
(a) Pointer data type(b) Activation Record
(c) Repeat -Until
(d) Coercion
Group-II
(p) Type Conversion(q) Dynamic Data Structure
(r) Recursion
(s) Nondeterministic loop
Marks 2
Refer to the given 3-address code sequence. This code sequence is split into basic blocks. The number of basic blocks is ________ . (Answer in integer)
$$\begin{aligned} & \text { 1001: } \mathrm{i}=1 \\ & \text { 1002: } \mathrm{j}=1 \\ & \text { 1003: } \mathrm{t} 1=10 \text { * } \mathrm{i} \\ & \text { 1004: } \mathrm{t} 2=\mathrm{t} 1+\mathrm{j} \\ & \text { 1005: t3 }=8^* \text { t2 } \\ & \text { 1006: } \mathrm{t} 4=\mathrm{t} 3-88 \\ & \text { 1007: a[t4] }=0.0 \\ & \text { 1008: j = j + } 1 \\ & \text { 1009: if } \mathrm{j}<=10 \text { goto } 1003 \\ & \text { 1010: } \mathrm{i}=\mathrm{i}+1 \\ & \text { 1011: if } \mathrm{i}<=10 \text { goto } 1002 \\ & \text { 1012: } \mathrm{i}=1 \\ & \text { 1013: } \mathrm{t} 5=\mathrm{i}-1 \\ & \text { 1014: t6 = 88 * t5 } \\ & \text { 1015: } \mathrm{a}[\mathrm{t} 6]=1.0 \\ & \text { 1016: } i=i+1 \\ & \text { 1017: if } \mathrm{i}<=10 \text { goto } 1013 \end{aligned}$$
Consider the following pseudo-code.
L1: t1 = -1
L2: t2 = 0
L3: t3 = 0
L4: t4 = 4 * t3
L5: t5 = 4 * t2
L6: t6 = t5 * M
L7: t7 = t4 + t6
L8: t8 = a[t7]
L9: if t8 <= max goto L11
L10: t1 = t8
L11: t3 = t3 + 1
L12: if t3 < M goto L4
L13: t2 = t2 + 1
L14: if t2 < N goto L3
L15: max = t1
Which one of the following options CORRECTLY specifies the number of basic blocks and the number of instructions in the largest basic block, respectively ?
Consider the control flow graph shown.
Which one of the following choices correctly lists the set of live variables at the exit point of each basic block?
Consider the following ANSI C code segment:
z = x + 3 + y -> f1 + y -> f2;
for (i = 0; i < 200; i = i + 2){
if (z > i) {
P = p + x + 3;
q = q + y -> f2;
} else {
p = p + y -> f2;
q = q + x + 3;
}
}
Assume that the variable y points to a struct (allocated on the heap) containing two fields f1 and f2, and the local variables x, y, z, p, g, and i are allotted registers. Common sub-expression elimination (CSE) optimization is applied on the code. The number of addition and dereference operations (of the form y -> f1 or y -> f2 ) in the optimized code, respectively, are:
For a statement S in a program, in the context of liveness analysis, the following sets are defined:
USE(S): the set of variables used in S
IN(S): the set of variables that are live at the entry of S
OUT(S): the set of variables that are live at the exit of S
Consider a basic block that consists of two statements, S1 followed by S2.
Which one of the following statements is correct?
(1) i = 1
(2) j = 1
(3) t1 = 5 ∗ i
(4) t2 = t1 + j
(5) t3 = 4 ∗ t2
(6) t4 = t3
(7) a[t4] = -1
(8) j = j + 1
(9) if j<=5 goto (3)
(10) i=i+1
(11) if i<5 goto (2)
The number of nodes and edges in the control-flow-graph constructed for the above code, respectively, are
i. There exists a statement $${S_j}$$ that uses x
ii. There is a path from $${S_i}$$ to $${S_j}$$ in the flow graph corresponding to the program
iii. The path has no intervening assignment to x including at $${S_i}$$ and $${S_j}$$
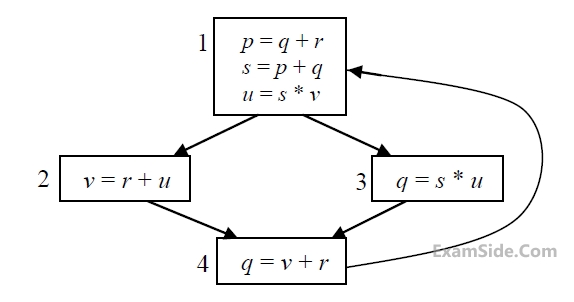
The variables which are live both at the statement in basic block 2 and at the statement in basic block 3 of the above control flow graph are
t0 = i * 1024
t1 = j * 32
t2 = k * 4
t3 = t1 + t0
t4 = t3 + t2
t5 = X[t4]
Which one of the following statements about the source code for the C program is CORRECT?Consider the following translation scheme.
$$\eqalign{ & S \to ER \cr & R \to *E\left\{ {pr{\mathop{\rm int}} ('*');} \right\}R\,|\,\varepsilon \cr & E \to F + E\left\{ {pr{\mathop{\rm int}} (' + ');} \right\}\,|\,F \cr & F \to S\,|\,id\,\left\{ {pr{\mathop{\rm int}} (id.value);} \right\} \cr} $$Here id is a token that represents an integer and id.value represents the corresponding integer value. For an input '2 * 3 + 4' this translation scheme prints
for (i = 0; i < n; i++)
{
for (j=0; j < n; j++)
{
if (i%2)
{
x += (4*j + 5*i);
y += (7 + 4*j);
}
}
}
Which one of the following is false?int main ( ) { /* Line 1 */
int I, N; /* Line 2 */
fro (I = 0, I < N, I++); /* Line 3 */
}
Identify the compiler's response about this line while creating the object-module Consider the syntax directed definition shown below.
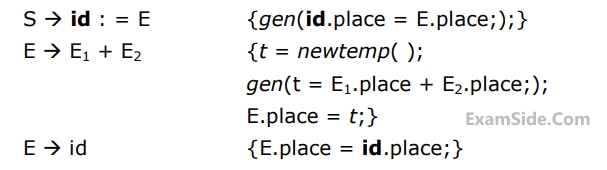
Here, gen is a function that generates the output code, and newtemp is a function that returns the name of a new temporary variable on every call. Assume that ti's are the temporary variable names generated by newtemp. For the statement 'X : = Y + Z', the 3-address code sequence generated by this definition is