Process Concepts and Cpu Scheduling · Operating Systems · GATE CSE
Marks 1
Consider a process P running on a CPU. Which one or more of the following events will always trigger a context switch by the OS that results in process P moving to a non-running state (e.g., ready, blocked)?
Which of the following process state transitions is/are NOT possible?
Which one or more of the following need to be saved on a context switch from one thread (T1) of a process to another thread (T2) of the same process?
Which one or more of the following CPU scheduling algorithms can potentially cause starvation?
I. A running process can move to ready state.
II. A ready process can move to ready state.
III. A blocked process can move to running state.
IV. A blocked process can move to ready state.
Which of the above statements are TRUE?
#include < unistd.h >
int main ()
{
int i ;
for (i=0; i<10; i++)
if (i%2 == 0) fork ( ) ;
return 0 ;
}
The total number of child processes created is _____.
fork $$\left( {\,\,\,} \right);$$
fork $$\left( {\,\,\,} \right);$$
fork $$\left( {\,\,\,} \right);$$
The total number of child processes created is
$$ * \,\,\,$$ Interrupt from $$CPU$$ temperature sensor (raises interrupt if $$CPU$$ temperature is too high)
$$ * \,\,\,$$ Interrupt from Mouse (raises interrupt if the mouse is moved or a button is pressed)
$$ * \,\,\,$$ Interrupt from keyboard (raises interrupt when a key is pressed or release)
$$ * \,\,\,$$ Interrupt from Hard Disk (raises interrupt when a disk read is completed)>/p>
Which one these will be handled at the HIGHEST priority?
Which of the following is TRUE?
$${\rm I}.$$ Shortest remaining time first scheduling may cause starvation
$${\rm II}.$$ Preemptive scheduling may cause starvation
$${\rm III}.$$ Round robin is better than $$FCFS$$ in terms of response time
Group-1
(P) Gang Scheduling
(Q) Rate Monotonic Scheduling
(R) Fair Share Scheduling
Group-2
(1) Guaranteed Scheduling
(2) Real-time Scheduling
(3) Thread Scheduling
Which one of the following statements is FALSE?
i) Context switch is faster with kernel- supported threads
ii) For user-level threads, a system call can block the entire process
iii) Kernel-supported threads can be scheduled independently
iv) Use-level threads are transparent to the kernel
Which of the above statements are true?
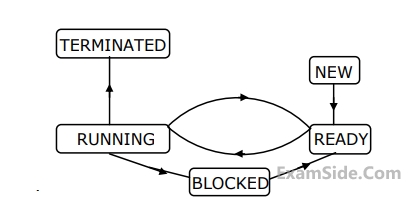
Marks 2
Consider a single processor system with four processes A, B, C, and D, represented as given below, where for each process the first value is its arrival time, and the second value is its CPU burst time.
A (0, 10), B (2, 6), C (4, 3), and D (6, 7).
Which one of the following options gives the average waiting times when preemptive Shortest Remaining Time First (SRTF) and Non-Preemptive Shortest Job First (NP-SJF) CPU scheduling algorithms are applied to the processes?
Consider four processes P, Q, R and S scheduled on a CPU as per round robin algorithm with a time quantum of 4 units. The processes arrive in the order P, Q, R, S, all at time t = 0. There is exactly one context switch from S to Q, exactly one context switch from R to Q, and exactly two context switches from Q to R. There is no context switch from S to P. Switching to a ready process after the termination of another process is also considered a context switch. Which one of the following is NOT possible as CPU burst time (in time units) of these processes?
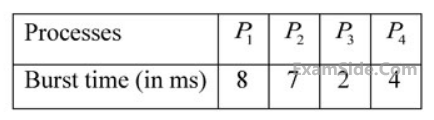
If the time quantum for RR is 4 ms, then the absolute value of the difference between the average turnaround times (in ms) of SJF and RR (round off to 2 decimal places) is _____.

These processes are run on a single processor using preemptive Shortest Remaining Time First scheduling algorithm. If the average waiting time of the processes is 1 millisecond, then the value of Z is _____.
Process | Arrival Time | Burst Time |
---|---|---|
P1 P2 P3 P4 |
0 3 7 8 |
10 6 1 3 |
The average turn around time of these processes is milliseconds.
Process | Arrival Time | Processing Time |
---|---|---|
A | 0 | 3 |
B | 1 | 6 |
D | 4 | 4 |
E | 6 | 2 |
Process | Arrival Time | Burst Time |
---|---|---|
P1 | 0 | 12 |
P2 | 2 | 4 |
P3 | 3 | 6 |
P4 | 8 | 5 |
The average waiting time (in milliseconds) of the processes is ________.
Process id | tc | tio |
---|---|---|
A | 100 ms | 500 ms |
B | 350 ms | 500 ms |
C | 200 ms | 500 ms |
The processes $$A, B,$$ and $$C$$ are started at times $$0, 5$$ and $$10$$ milliseconds respectively, in a pure time sharing system (round robin scheduling) that uses a time slice of $$50$$ milliseconds. The time in milliseconds at which process $$C$$ would complete its first $${\rm I}/O$$ operation is __________.
Process Name | Arrival Time | Execution Time |
---|---|---|
A | 0 | 6 |
B | 3 | 2 |
C | 5 | 4 |
D | 7 | 6 |
E | 10 | 3 |
Using the $$shortest$$ $$remaining$$ $$time$$ $$first$$ scheduling algorithm, the average process turnaround time (in $$msec$$) is _______.
Process | Arrival Time | Time Units Required |
---|---|---|
P1 | 0 | 5 |
P2 | 1 | 7 |
P3 | 3 | 4 |
The completion order of the $$3$$ processes under the policies $$FCFS$$ and $$RR2$$ (round robin scheduling with $$CPU$$ quantum of $$2$$ time units) are
Process | Arrival Time | Burst Time |
---|---|---|
P0 | 0 ms | 9 ms |
P1 | 1 ms | 4 ms |
P2 | 2 ms | 9 ms |
The pre-emptive shortest job first scheduling algorithm is used. Scheduling is carried out only a arrival or completion of processes. What is the average waiting time for the three processes?
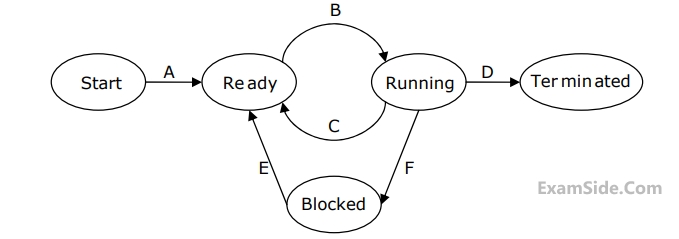
Now consider the following statements:
$$1.\,\,\,$$ If a process makes a transition $$D,$$ it would result in another process making transition $$A$$ immediately.
$$2.\,\,\,$$ $$A$$ process $${P_2}$$ in blocked state can make transition $$E$$ while another process $${P_1}$$ is in running state.
$$3.\,\,\,$$ The $$OS$$ uses preemptive scheduling.
$$4.\,\,\,$$ The $$OS$$ uses non-preemptive scheduling.
Which of the above statements are TRUE?
for (i = 0; i < n; i + +) fork ( );
The total number of child processes created is$${\rm I}.\,\,\,$$ It is useful in creating self-relocating code
$${\rm II}.\,\,\,$$ If it is included in an Instruction Set Architecture, then an additional $$ALU$$ is required for effective address calculation.
$${\rm III}.\,\,\,$$ The amount of increment depends on the size of the data item accessed
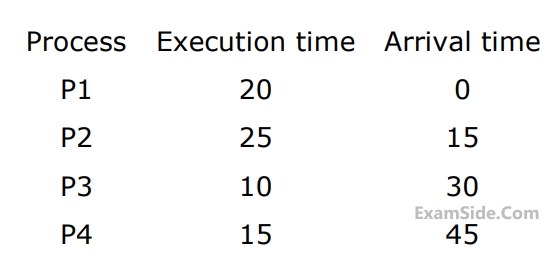
What is the total waiting time for process $$P2?$$
if (fork() == 0)
{
a = a + 5;
printf("%d, %d \n", a, &a);
}
else
{
a = a - 5;
printf ("%d, %d \n", a, &a);
}
Let $$u,v$$ be the values printed by the parent process, and $$x,y$$ be the values printed by the child process. Which one of the following is TRUE?
What is the average turnaround time for these processes with the preemptive shortest remaining processing time first $$(SRTF)$$ algorithm?
$$(a).$$ More than one program may be loaded into main memory at the same time for execution.
$$(b).$$ If a program waits for certain events such as $$I/O$$, another program is immediately scheduled for execution.
$$(c).$$ If the execution of a program terminates, another program is immediately scheduled for execution.
(i) each process is in one of the five states: created, ready, running, blocked (i.e. sleep or wait), or terminated, and
(ii) only non-preemptive scheduling is used by the $$OS.$$ Label the transitions appropriately.
Job Id | CPU Burst Time |
---|---|
p | 4 |
q | 1 |
r | 8 |
s | 1 |
t | 2 |
The jobs are assumed to have arrived at time $${0^ + }$$ and in the order $$p,q,r,s,t.$$ Calculate the departure time (completion time) for job $$p$$ if scheduling is round robin with time slice$$1.$$
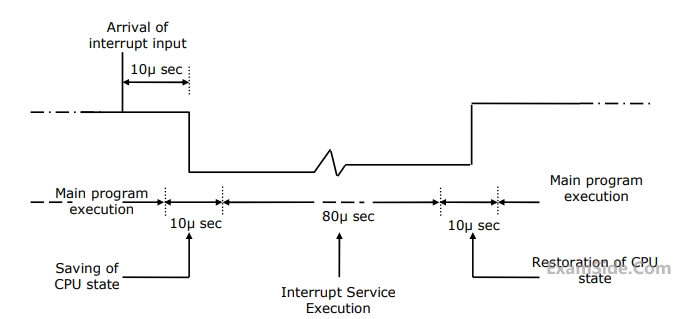
Given that an interrupt input arrives every $$1$$ $$msec,$$ what is the percentage of the total time that the $$CPU$$ devotes for the main program execution.
(a) Turn around time. (b) Waiting time.