Consider the C function foo and the binary tree shown.
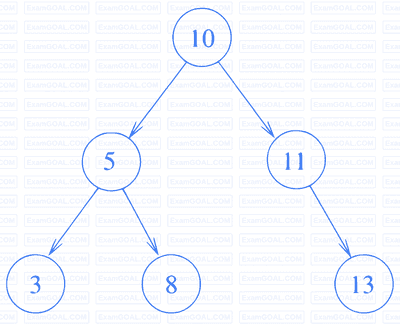
typedef struct node {
int val;
struct node *left, *right;
} node;
int foo(node *p) {
int retval;
if (p == NULL)
return 0;
else {
retval = p->val + foo(p->left) + foo(p->right);
printf("%d ", retval);
return retval;
}
}
When foo is called with a pointer to the root node of the given binary tree, what will it print?
Consider a sequence a of elements $$a_0=1,a_1=5,a_2=7,a_3=8,a_4=9$$, and $$a_5=2$$. The following operations are performed on a stack S and a queue Q, both of which are initially empty.
I: push the elements of a from a$$_0$$ to a$$_5$$ in that order into S.
II: enqueue the elements of a from a$$_0$$ to a$$_5$$ in that order into Q.
III: pop an element from S.
IV: dequeue an element from Q.
V: pop an element from S.
VI: dequeue an element from Q.
VII: dequeue an element from Q and push the same
VIII: Repeat operation VII three times.
IX: pop an element from S.
X: pop an element from S.
The top element of S after executing the above operations is ____________.
Which one of the options given below refers to the degree (or arity) of a relation in relational database systems?
Consider the following table named Student in a relational database. The primary key of this table is rollNum.
Student
rollNum | name | gender | marks |
---|---|---|---|
1 | Naman | M | 62 |
2 | Aliya | F | 70 |
3 | Aliya | F | 80 |
4 | James | M | 82 |
5 | Swati | F | 65 |
The SQL query below is executed on this database.
SELECT *
FROM Student
WHERE gender = 'F' AND
marks > 65;
The number of rows returned by the query is _____________.