GATE CSE
Given an integer array of size $N$, we want to check if the array is sorted (in either ascending or descending order). An algorithm solves this problem by making a single pass through the array and comparing each element of the array only with its adjacent elements. The worst-case time complexity of this algorithm is
Consider the following recurrence relation:
$$T(n) = \begin{cases} \sqrt{n} T(\sqrt{n}) + n & \text{for } n \ge 1, \\ 1 & \text{for } n = 1. \end{cases}$$
Which one of the following options is CORRECT?
Which of the following is/are Bottom-Up Parser(s)?
Consider the following syntax-directed definition (SDD).
S → DHTU | { S.val = D.val + H.val + T.val + U.val; } |
D → “M” D1 | { D.val = 5 + D1.val; } |
D → ε | { D.val = –5; } |
H → “L” H1 | { H.val = 5 * 10 + H1.val; } |
H → ε | { H.val = –10; } |
T → “C” T1 | { T.val = 5 * 100 + T1.val; } |
T → ε | { T.val = –5; } |
U → “K” | { U.val = 5; } |
Consider the following grammar $G$, with $S$ as the start symbol. The grammar $G$ has three incomplete productions denoted by (1), (2), and (3).
$$S \rightarrow d a T \mid \underline{\ (1)}$$
$$T \rightarrow a S \mid b T \mid \ \underline{(2)}$$
$$R \rightarrow \underline{(3)} \mid \epsilon$$
The set of terminals is $\{a, b, c, d, f\}$. The FIRST and FOLLOW sets of the different non-terminals are as follows.
FIRST($S$) = $\{c, d, f\}$, FIRST($T$) = $\{a, b, \epsilon\}$, FIRST($R$) = $\{c, \epsilon\}$
FOLLOW($S$) = FOLLOW($T$) = $\{c, f, \#\}$, FOLLOW($R$) = $\{f\}$
Which one of the following options CORRECTLY fills in the incomplete productions?
Consider the following pseudo-code.
L1: t1 = -1
L2: t2 = 0
L3: t3 = 0
L4: t4 = 4 * t3
L5: t5 = 4 * t2
L6: t6 = t5 * M
L7: t7 = t4 + t6
L8: t8 = a[t7]
L9: if t8 <= max goto L11
L10: t1 = t8
L11: t3 = t3 + 1
L12: if t3 < M goto L4
L13: t2 = t2 + 1
L14: if t2 < N goto L3
L15: max = t1
Which one of the following options CORRECTLY specifies the number of basic blocks and the number of instructions in the largest basic block, respectively ?
A user starts browsing a webpage hosted at a remote server. The browser opens a single TCP connection to fetch the entire webpage from the server. The webpage consists of a top-level index page with multiple embedded image objects. Assume that all caches (e.g., DNS cache, browser cache) are all initially empty. The following packets leave the user's computer in some order.
(i) HTTP GET request for the index page
(ii) DNS request to resolve the web server's name to its IP address
(iii) HTTP GET request for an image object
(iv) TCP SYN to open a connection to the web server
Which one of the following is the CORRECT chronological order (earliest in time to latest) of the packets leaving the computer?
TCP client P successfully establishes a connection to TCP server Q. Let $N_P$ denote the sequence number in the SYN sent from P to Q. Let $N_Q$ denote the acknowledgement number in the SYN ACK from Q to P. Which of the following statements is/are CORRECT?
Which of the following fields is/are modified in the IP header of a packet going out of a network address translation (NAT) device from an internal network to an external network?
Consider a network path P—Q—R between nodes P and R via router Q. Node P sends a file of size $10^6$ bytes to R via this path by splitting the file into chunks of $10^3$ bytes each. Node P sends these chunks one after the other without any wait time between the successive chunk transmissions. Assume that the size of extra headers added to these chunks is negligible, and that the chunk size is less than the MTU.
Each of the links P—Q and Q—R has a bandwidth of $10^6$ bits/sec, and negligible propagation latency. Router Q immediately transmits every packet it receives from P to R, with negligible processing and queueing delays. Router Q can simultaneously receive on link P—Q and transmit on link Q—R.
Assume P starts transmitting the chunks at time $t = 0$.
Which one of the following options gives the time (in seconds, rounded off to 3 decimal places) at which R receives all the chunks of the file?
Consider the entries shown below in the forwarding table of an IP router. Each entry consists of an IP prefix and the corresponding next hop router for packets whose destination IP address matches the prefix. The notation “/N” in a prefix indicates a subnet mask with the most significant N bits set to 1.
Prefix | Next hop router |
---|---|
10.1.1.0/24 | R1 |
10.1.1.128/25 | R2 |
10.1.1.64/26 | R3 |
10.1.1.192/26 | R4 |
This router forwards 20 packets each to 5 hosts. The IP addresses of the hosts are 10.1.1.16, 10.1.1.72, 10.1.1.132, 10.1.1.191, and 10.1.1.205 . The number of packets forwarded via the next hop router R2 is _______
Consider sending an IP datagram of size 1420 bytes (including 20 bytes of IP header) from a sender to a receiver over a path of two links with a router between them. The first link (sender to router) has an MTU (Maximum Transmission Unit) size of 542 bytes, while the second link (router to receiver) has an MTU size of 360 bytes.
The number of fragments that would be delivered at the receiver is ______________
Which one of the following statements is FALSE?
Consider a 5-stage pipelined processor with Instruction Fetch (IF), Instruction Decode (ID), Execute (EX), Memory Access (MEM), and Register Writeback (WB) stages. Which of the following statements about forwarding is/are CORRECT?
Consider two set-associative cache memory architectures: WBC, which uses the write back policy, and WTC, which uses the write through policy. Both of them use the LRU (Least Recently Used) block replacement policy. The cache memory is connected to the main memory. Which of the following statements is/are TRUE?
Consider a 512 GB hard disk with 32 storage surfaces. There are 4096 sectors per track and each sector holds 1024 bytes of data. The number of cylinders in the hard disk is ______
The baseline execution time of a program on a 2 GHz single core machine is 100 nanoseconds (ns). The code corresponding to 90% of the execution time can be fully parallelized. The overhead for using an additional core is 10 ns when running on a multicore system. Assume that all cores in the multicore system run their share of the parallelized code for an equal amount of time.
$$ \text { The number of cores that minimize the execution time of the program is _______. } $$A given program has 25% load/store instructions. Suppose the ideal CPI (cycles per instruction) without any memory stalls is 2. The program exhibits 2% miss rate on instruction cache and 8% miss rate on data cache. The miss penalty is 100 cycles. The speedup (rounded off to two decimal places) achieved with a perfect cache (i.e., with NO data or instruction cache misses) is ______
Consider the operator precedence and associativity rules for the integer arithmetic operators given in the table below.
Operator | Precedence | Associativity |
---|---|---|
+ | Highest | Left |
− | High | Right |
* | Medium | Right |
/ | Low | Right |
The value of the expression $3 + 1 + 5 * 2 / 7 + 2 − 4 − 7 − 6 / 2$ as per the above rules is _______
An array $[82, 101, 90, 11, 111, 75, 33, 131, 44, 93]$ is heapified. Which one of the following options represents the first three elements in the heapified array?
Consider a binary min-heap containing 105 distinct elements. Let k be the index (in the underlying array) of the maximum element stored in the heap. The number of possible values of k is
Let G be a directed graph and T a depth first search (DFS) spanning tree in G that is rooted at a vertex v. Suppose T is also a breadth first search (BFS) tree in G, rooted at v. Which of the following statements is/are TRUE for every such graph G and tree T?
Let S be the specification : "Instructors teach courses. Students register for courses. Courses are allocated classrooms. Instructors guide students." Which one of the following ER diagrams CORRECTLY represents S?
In a B+ tree, the requirement of at least half-full (50%) node occupancy is relaxed for which one of the following cases?
Which of the following statements about a relation $R$ in first normal form (1NF) is/are TRUE?
Consider the following two relations, R(A, B) and S(A, C):
R | |
---|---|
A | B |
10 | 20 |
20 | 30 |
30 | 40 |
30 | 50 |
50 | 95 |
S | |
---|---|
A | C |
10 | 90 |
30 | 45 |
40 | 80 |
The total number of tuples obtained by evaluating the following expression
$$ \sigma_{B < C}(R \bowtie_{R.A = S.A} S) $$
is _________
The symbol → indicates functional dependency in the context of a relational database. Which of the following options is/are TRUE?
Consider the following read-write schedule $S$ over three transactions $T_{1}$, $T_{2}$, and $T_{3}$, where the subscripts in the schedule indicate transaction IDs:
$S: r_{1}(z); w_{1}(z); r_{2}(x); r_{3}(y); w_{3}(y); r_{2}(y); w_{2}(x); w_{2}(y);$
Which of the following transaction schedules is/are conflict equivalent to $S$?
Consider a system that uses 5 bits for representing signed integers in 2’s complement format. In this system, two integers A and B are represented as A=01010 and B=11010. Which one of the following operations will result in either an arithmetic overflow or an arithmetic underflow?
Consider the circuit shown below where the gates may have propagation delays. Assume that all signal transitions occur instantaneously and that wires have no delays. Which of the following statements about the circuit is/are CORRECT?

Consider a Boolean expression given by $F(X, Y, Z) = \Sigma(3,5,6,7)$.
Which of the following statements is/are CORRECT?
Consider a digital logic circuit consisting of three 2-to-1 multiplexers M1, M2, and M3 as shown below. X1 and X2 are inputs of M1. X3 and X4 are inputs of M2. A, B, and C are select lines of M1, M2, and M3, respectively.
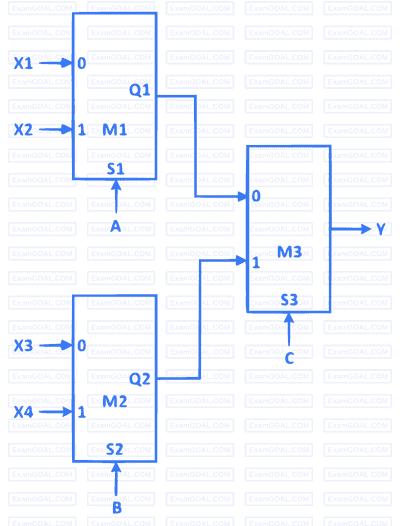
For an instance of inputs X1=1, X2=1, X3=0, and X4=0, the number of combinations of A, B, C that give the output Y=1 is ______________
Let $f : \mathbb{R} \rightarrow \mathbb{R}$ be a function such that $f(x) = \max \{x, x^3\}, x \in \mathbb{R}$, where $\mathbb{R}$ is the set of all real numbers. The set of all points where $f(x)$ is NOT differentiable is
The product of all eigenvalues of the matrix $\begin{bmatrix} 1 & 2 & 3 \\ 4 & 5 & 6 \\ 7 & 8 & 9 \end{bmatrix}$ is
Consider a permutation sampled uniformly at random from the set of all permutations of {1, 2, 3, ..., n} for some n ≥ 4. Let X be the event that 1 occurs before 2 in the permutation, and Y the event that 3 occurs before 4. Which one of the following statements is TRUE?
Let A and B be two events in a probability space with $P(A) = 0.3$, $P(B) = 0.5$, and $P(A \cap B) = 0.1$. Which of the following statements is/are TRUE?
Let $A$ and $B$ be non-empty finite sets such that there exist one-to-one and onto functions (i) from $A$ to $B$ and (ii) from $A \times A$ to $A \cup B$. The number of possible values of $|A|$ is _______
The number of spanning trees in a complete graph of 4 vertices labelled A, B, C, and D is __________
Let A be any n x m matrix, where m > n. Which of the following statements is/are TRUE about the system of linear equations Ax = 0?
The chromatic number of a graph is the minimum number of colours used in a proper colouring of the graph. Let $G$ be any graph with $n$ vertices and chromatic number $k$. Which of the following statements is/are always TRUE?
The number of edges present in the forest generated by the DFS traversal of an undirected graph G with 100 vertices is 40. The number of connected components in G is ________
A bag contains 10 red balls and 15 blue balls. Two balls are drawn randomly without replacement. Given that the first ball drawn is red, the probability (rounded off to 3 decimal places) that both balls drawn are red is ________
Which of the following statements about threads is/are TRUE?
Which of the following process state transitions is/are NOT possible?
Consider the following two threads T1 and T2 that update two shared variables a and b. Assume that initially $a = 1$ and $b = 1$. Though context switching between threads can happen at any time, each statement of T1 or T2 is executed atomically without interruption.
T1:
$a = a + 1$;
$b = b + 1$;
T2:
$b = 2 * b$;
$a = 2 * a$;
Which one of the following options lists all the possible combinations of values of a and b after both T1 and T2 finish execution?
Consider the following code snippet using the fork()
and wait()
system calls. Assume that the code compiles and runs correctly, and that the system calls run successfully without any errors.
int x = 3;
while(x > 0) {
fork();
printf("hello");
wait(NULL);
x--;
}
The total number of times the printf
statement is executed is _______
Consider a memory management system that uses a page size of 2 KB. Assume that both the physical and virtual addresses start from 0. Assume that the pages 0, 1, 2, and 3 are stored in the page frames 1, 3, 2, and 0, respectively. The physical address (in decimal format) corresponding to the virtual address 2500 (in decimal format) is ________
Consider the following C program:
#include <stdio.h>
int main() {
int a = 6;
int b = 0;
while(a < 10) {
a = a / 12 + 1;
a += b;}
printf("%d", a);
return 0;}
Which one of the following statements is CORRECT?
Consider the following C program:
#include <stdio.h>
void fX();
int main() {
fX();
return 0;}
void fX() {
char a;
if ((a = getchar()) != '\n')
fX();
if (a != '\n')
putchar(a);}
Assume that the input to the program from the command line is 1234 followed by a newline character. Which one of the following statements is CORRECT?
Consider the following C function definition.
int f(int x, int y) {
for (int i=0; i<y; i++) {
x=x+x+y;
}
return x;
}
Which of the following statements is/are TRUE about the above function?
Let $L_1, L_2$ be two regular languages and $L_3$ a language which is not regular. Which of the following statements is/are always TRUE?
Consider the 5-state DFA $M$ accepting the language $L(M) \subseteq (0+1)^*$ shown below. For any string $w \in (0+1)^*$ let $n_0(w)$ be the number of 0's in $w$ and $n_1(w)$ be the number of 1's in $w$.
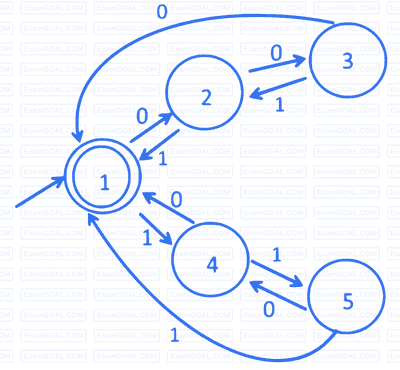
Which of the following statements is/are FALSE?
Let G = (V, Σ, S, P) be a context-free grammar in Chomsky Normal Form with Σ = { a, b, c } and V containing 10 variable symbols including the start symbol S. The string w = a30b30c30 is derivable from S. The number of steps (application of rules) in the derivation S ⟹ w is _______
Consider the following two regular expressions over the alphabet {0,1} :
$$r = 0^* + 1^*$$
$$s = 01^* + 10^*$$
The total number of strings of length less than or equal to 5, which are neither in r nor in s, is ________
General Aptitude
Consider the following sample of numbers:
9, 18, 11, 14, 15, 17, 10, 69, 11, 13
The median of the sample is
The number of coins of ₹1, ₹5, and ₹10 denominations that a person has are in the ratio 5:3:13. Of the total amount, the percentage of money in ₹5 coins is
If ‘→’ denotes increasing order of intensity, then the meaning of the words [dry → arid → parched] is analogous to [diet → fast → ________ ].
Which one of the given options is appropriate to fill the blank?
If two distinct non-zero real variables $x$ and $y$ are such that $(x + y)$ is proportional to $(x - y)$ then the value of $\frac{x}{y}$
For positive non-zero real variables $p$ and $q$, if
$\log \left(p^2 + q^2\right) = \log p + \log q + 2 \log 3$,
then, the value of $\frac{p^4 + q^4}{p^2 q^2}$ is
In the given text, the blanks are numbered (i)–(iv). Select the best match for all the blanks.
Steve was advised to keep his head _____ (i) _____ before heading _____ (ii) _____ to bat; for, while he had a head _____ (iii) _____ batting, he could only do so with a cool head _____ (iv) _____ his shoulders.
A rectangular paper sheet of dimensions 54 cm × 4 cm is taken. The two longer edges of the sheet are joined together to create a cylindrical tube. A cube whose surface area is equal to the area of the sheet is also taken.
Then, the ratio of the volume of the cylindrical tube to the volume of the cube is
The pie chart presents the percentage contribution of different macronutrients to a typical 2,000 kcal diet of a person.
Macronutrient energy contribution
The typical energy density (kcal/g) of these macronutrients is given in the table:
Macronutrient | Energy density (kcal/g) |
---|---|
Carbohydrates | 4 |
Proteins | 4 |
Unsaturated fat | 9 |
Saturated fat | 9 |
Trans fat | 9 |
The total fat (all three types), in grams, this person consumes is
A rectangular paper of 20 cm × 8 cm is folded 3 times. Each fold is made along the line of symmetry, which is perpendicular to its long edge. The perimeter of the final folded sheet (in cm) is
The least number of squares to be added in the figure to make AB a line of symmetry is
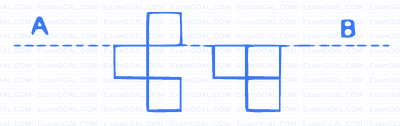