GATE CSE
int fun1 (int n) {
int i, j, k, p, q = 0;
for (i = 1; i < n; ++i)
{
p = 0;
for (j = n; j > 1; j = j/2)
++p;
for (k = 1; k < p; k = k * 2)
++q;
}
return q;
}
Which one of the following most closely approximates the return value of the function fun1?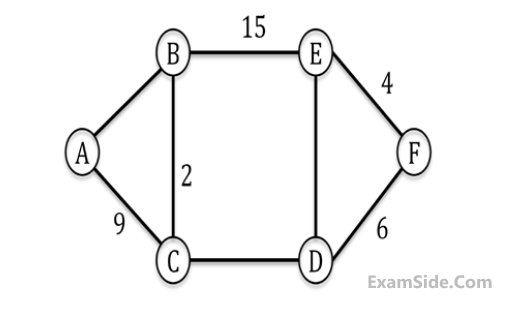
List 1
(P) Prim’s algorithm for minimum spanning tree(Q) Floyd-Warshall algorithm for all pairs shortest paths
(R) Mergesort
(S) Hamiltonian circuit
List 2
(i) Backtracking(ii) Greedy method
(iii) Dynamic programming
(iv) Divide and conquer
Array Index | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
---|---|---|---|---|---|---|---|---|---|
Value | 40 | 30 | 20 | 10 | 15 | 16 | 17 | 8 | 4 |
Now consider that a value 35 is inserted into this heap. After insertion, the new heap is
i. There exists a statement $${S_j}$$ that uses x
ii. There is a path from $${S_i}$$ to $${S_j}$$ in the flow graph corresponding to the program
iii. The path has no intervening assignment to x including at $${S_i}$$ and $${S_j}$$
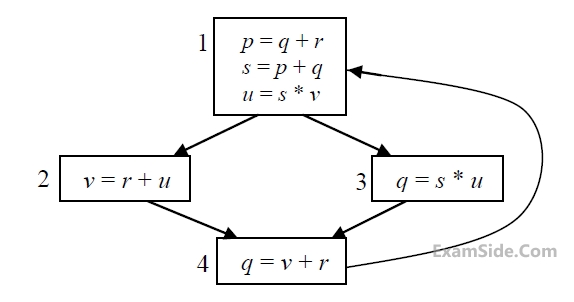
The variables which are live both at the statement in basic block 2 and at the statement in basic block 3 of the above control flow graph are
I. If the sequence number of a segment is m, then the sequence number of the subsequent segment is always m + 1.
II. If the estimated round trip time at any given point of time is t sec, the value of the retransmission timeout is always set to greater than or equal to t sec.
III. The size of the advertised window never changes during the course of the TCP connection.
IV. The number of unacknowledged bytes at the sender is always less than or equal to the advertised window
(S1) A memory operand
(S2) A processor register
(S3) An implied accumulator register
I. 3, 5, 7, 8, 15, 19, 25
II. 5, 8, 9, 12, 10, 15, 25
III. 2, 7, 10, 8, 14, 16, 20
IV. 4, 6, 7, 9 18, 20, 25
Consider an Entity-Relationship (ER) model in which entity sets E1 and E2 are connected by an m : n relationship R12. E1 and E3 are connected by a 1 : n (1 on the side of E1 and n on the side of E3) relationship R13.
E1 has two single-valued attributes a11 and a12 of which a11 is the key attribute. E2 has two single-valued attributes a21 and a22 of which a21 is the key attribute. E3 has two single-valued attributes a31 and a32 of which a31 is the key attribute. The relationships do not have any attributes.
If a relational model is derived from the above ER model, then the minimum number of relations that would be generated if all the relations are in 3NF is _______.
Consider the following relation:
Student
Roll_No | Student_Name |
---|---|
1 | Raj |
2 | Rohit |
3 | Raj |
Performance
Roll_No | Course | Marks |
---|---|---|
1 | Math | 80 |
1 | English | 70 |
2 | Math | 75 |
3 | English | 80 |
2 | Physics | 65 |
3 | Math | 80 |
Consider the following SQL query.
SELECT S.Student_Name, Sum(P.Marks)
FROM Student S, Performance P
WHERE S.Roll_No= P.Roll_No
GROUP BY S.STUDENT_Name
The numbers of rows that will be returned by the SQL query is___________.Consider the operations
$$f\left( {x,y,z} \right) = X'YZ + XY' + Y'Z'$$ and$$g\left( {x,y,z} \right) = X'YZ + X'YZ' + XY$$.
Which one of the following is correct?
The binary operator $$ \ne $$ is defined by the following truth table.
p | q | p$$ \ne $$q |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Which one of the following is true about the binary operator $$ \ne $$?
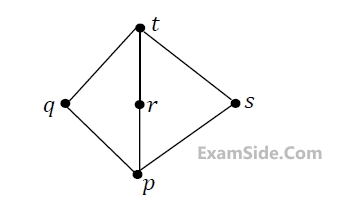
$${\rm I}.$$ $$\,\,\,\,\,\,$$ $$p+m+c=27/20$$
$${\rm I}{\rm I}.$$ $$\,\,\,\,\,\,$$ $$p+m+c=13/20$$
$${\rm I}{\rm I}{\rm I}.$$ $$\,\,\,\,\,\,$$ $$\left( p \right) \times \left( m \right) \times \left( c \right) = 1/10$$
$$A = \left( {\matrix{ 1 & 4 \cr b & a \cr } } \right)$$
I. $$\phi \in {2^A}$$
II. $$\phi \subseteq {2^A}$$
III. $$\left\{ {5,\left\{ 6 \right\}} \right\} \in {2^A}$$
IV. $$\left\{ {5,\left\{ 6 \right\}} \right\} \subseteq {2^A}$$
45, 20, 90, 10, 50, 60, 80, 25, 70.
Assume that the initial position of the R/W head is on track 50. The additional distance that will be traversed by the R/W head when the Shortest Seek Time First (SSTF) algorithm is used compared to the SCAN (Elevator) algorithm (assuming that SCAN algorithm moves towards 100 when it starts execution) is____________ tracks.P1( ) {
C = B – 1;
B = 2 * C;
}
P2( ) {
D = 2 * B;
B = D - 1;
}
The number of distinct values that B can possibly take after the execution is___________.int main () {
unsigned int x[4][3] =
{{1,2,3},{4,5,6},{7,8,9},{10,11,12}};
printf(“%u, %u, %u”, x+3, *(x+3), *(x+2)+3);
}
begin
q := 0
r := x
while r ≥ y do
begin
r := r - y
q := q + 1
end
end
The post condition that needs to be satisfied after the program terminates is
void f1(int a, int b) {
int c;
c=a; a=b; b=c;
}
void f2(int *a, int *b) {
int c;
c=*a; *a=*b; *b=c;
}
int main(){
int a=4, b=5, c=6;
f1(a,b);
f2(&b, &c);
printf(“%d”,c-a-b);
}
Match the following:
List I | List II |
---|---|
(P) Condition coverage | (i) Black-box testing |
(Q) Equivalence class partitioning | (ii) System testing |
(R) Volume testing | (iii) White-box testing |
(S) Alpha testing | (iv) Performance testing |
while(first <= last)
{
if (array[middle] < search)
first = middle + 1;
else if (array[middle] == search)
found = TRUE;
else last = middle - 1;
middle = (first + last)/2;
}
if (first > last) notPresent = TRUE;
The cyclomatic complexity of the program segment is ________.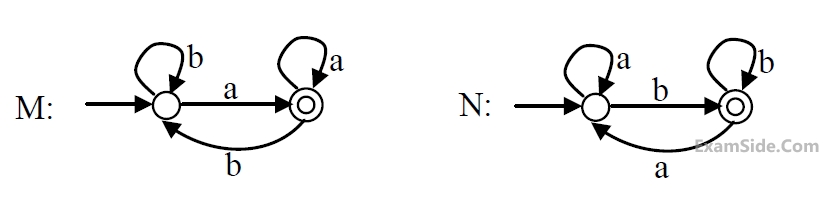
Consider the DFAs M and N given above. The number of states in a minimal DFA that accepts the language L(M) ∩ L(N) is___________.
Which one of the following sequences must follow the string 101100 so that the overall string is accepted by the automaton?
For any two languages L1 and L2 such that L1 is context-free and L2 is recursively enumerable but not recursive, which of the following is/are necessarily true?
I. $${\overline L _1}$$ (complement of L1) is recursiveII. $${\overline L _2}$$ (complement of L2) is recursive
III. $${\overline L _1}$$ is context-free
IV. $${\overline L _1} \cup {L_2}$$ is recursively enumerable
I. XML overcomes the limitations in HTML to support a structured way of organizing content.
II. XML specification is not case sensitive while HTML specification is case sensitive.
III. XML supports user defined tags while HTML uses pre-defined tags.
IV. XML tags need not be closed while HTML tags must be closed.
General Aptitude
Statement:
There has been a significant drop in the water level in the lakes supplying water to the city.
Course of action:
(I) The water supply authority should impose a partial cut in supply to tackle the situation.
(II) The government should appeal to all the residents through mass media for minimal
use of water.
(III) The government should ban the water supply in lower areas.
The number of students in a class who have answered correctly, wrongly, or not attempted each question in an exam, are listed in the table below. The marks for each question are also listed. There is no negative or partial marking.
Q No | Marks | Answered Correctly |
Answered Wrongly |
Not Attempted |
---|---|---|---|---|
1 | 2 | 21 | 17 | 6 |
2 | 3 | 15 | 27 | 2 |
3 | 1 | 11 | 29 | 4 |
4 | 2 | 23 | 18 | 3 |
5 | 5 | 31 | 12 | 1 |
What is the average of the marks obtained by the class in the examination?
If two floors in a certain building are 9 feet apart, how many steps are there in a set of stairs that extends from the first floor to the second floor of the building?
Statements:(I) Each step is $${3 \over 4}$$ foot high.
(II) Each step is 1 foot wide.
The chain snatchers took to their heels when the police party arrived.
She enjoyed herself immensely at the party.
$$\left( I \right)\,p + m + c = {{27} \over {20}}$$
$$\left( {II} \right)\,p + m + c = {{13} \over {20}}$$
$$\left( {III} \right)\,\left( p \right) \times \left( m \right) \times \left( c \right) = {1 \over {10}}$$
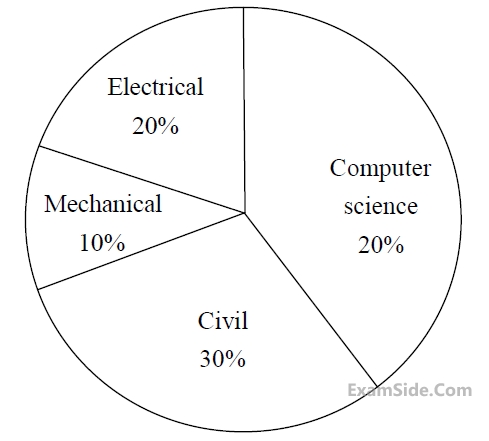