Let A be a priority queue for maintaining a set of elements. Suppose A is implemented using a max-heap data structure. The operation EXTRACT-MAX(A) extracts and deletes the maximum element from A. The operation INSERT(A, key) inserts a new element key in A. The properties of a max-heap are preserved at the end of each of these operations.
When A contains n elements, which one of the following statements about the worst case running time of these two operations is TRUE?
Consider the C function foo and the binary tree shown.
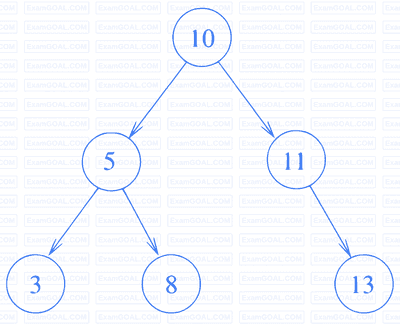
typedef struct node {
int val;
struct node *left, *right;
} node;
int foo(node *p) {
int retval;
if (p == NULL)
return 0;
else {
retval = p->val + foo(p->left) + foo(p->right);
printf("%d ", retval);
return retval;
}
}
When foo is called with a pointer to the root node of the given binary tree, what will it print?
Consider a sequence a of elements $$a_0=1,a_1=5,a_2=7,a_3=8,a_4=9$$, and $$a_5=2$$. The following operations are performed on a stack S and a queue Q, both of which are initially empty.
I: push the elements of a from a$$_0$$ to a$$_5$$ in that order into S.
II: enqueue the elements of a from a$$_0$$ to a$$_5$$ in that order into Q.
III: pop an element from S.
IV: dequeue an element from Q.
V: pop an element from S.
VI: dequeue an element from Q.
VII: dequeue an element from Q and push the same
VIII: Repeat operation VII three times.
IX: pop an element from S.
X: pop an element from S.
The top element of S after executing the above operations is ____________.
Which one of the options given below refers to the degree (or arity) of a relation in relational database systems?