GATE CSE
The program below uses six temporary variables a, b, c, d, e, f.
a = 1
b = 10
c = 20
d = a + b
e = c + d
f = c + e
b = c + e
e = b + f
d = 5 + e
return d + f
Assuming that all operations take their operands from registers, what is the minimum number of registers needed to execute this program without spilling?
Consider a network with 6 routers R1 to R6 connected with links having weights as shown in the following diagram
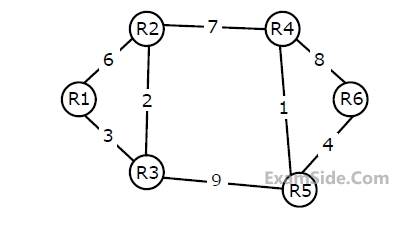
All the routers use the distance vector based routing algorithm to update their routing tables. Each router starts with its routing table initialized to contain an entry for each neighbour with the weight of the respective connecting link. After all the routing tables stabilize, how many links in the network will never be used for carrying any data?
Consider a network with 6 routers R1 to R6 connected with links having weights as shown in the following diagram
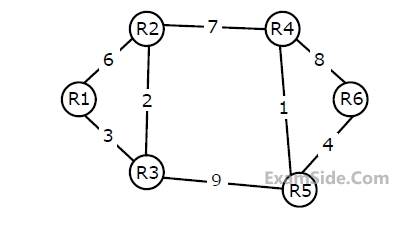

When there is a miss in both $$L1$$ cache and $$L2$$ cache, first a block is transferred from main memory to $$L2$$ cache, and then a block is transferred from $$L2$$ cache to $$L1$$ cache.
What is the total time taken for these transfers?

When there is a miss in $$L1$$ cache and a hit in $$L2$$ cache, a block is transferred from $$L2$$ cache to $$L1$$ cache. What is the time taken for this transfer?
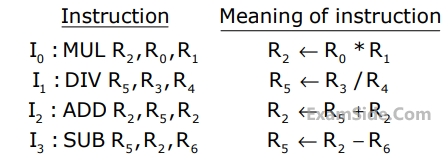
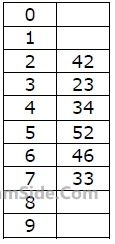
typedef struct node {
int value;
struct node *next;
} Node;
Node *move_to_front(Node *head) {
Node *p, *q;
if ((head = = NULL: || (head->next = = NULL)) return head;
q = NULL; p = head;
while (p-> next !=NULL) {
q=P;
p=p->next;
}
return head;
}
Choose the correct alternative to replace the blank line. 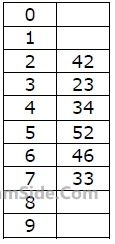
A relational schema for a train reservation database is given below:
Passenger ( pid, pname, age)
Reservation (pid, cass, tid)
Table: Passenger
pid | pname | age |
---|---|---|
0 | 'Sachin' | 65 |
1 | 'Rahul' | 66 |
2 | 'Sourav' | 67 |
3 | 'Anil' | 69 |
Table: Reservation
pid | class | tid |
---|---|---|
0 | 'AC' | 8200 |
1 | 'AC' | 8201 |
2 | 'SC' | 8201 |
5 | 'AC' | 8203 |
1 | 'SC' | 8204 |
3 | 'AC' | 8202 |
What pids are returned by the following SQL query for the above instance of the tables?
SELECT pid
FROM Reservation
WHERE class 'AC' AND
EXISTS (SELECT *
FROM Passenger
WHERE age > 65
AND Passenger.pid = Reservation.pid);
The relation R contains 200 tuples and the relation S contains 100 tuples. What is the maximum number of tuples possible in the natural join R $$\Join$$ S?
I. 2-phase locking
II. Time-stamp ordering
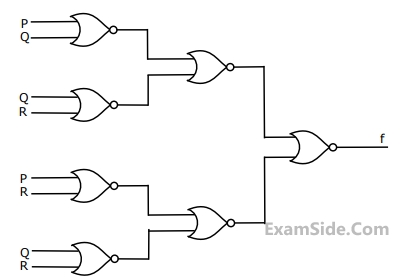
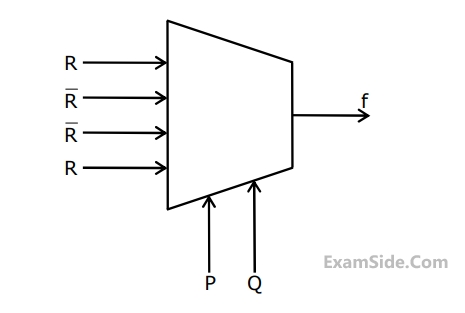
$${\rm I}.$$$$\,\,\,\,\,7,6,5,4,4,3,2,1$$
$${\rm I}{\rm I}.$$$$\,\,\,\,\,6,6,6,6,3,3,2,2$$
$${\rm I}{\rm I}{\rm I}.$$$$\,\,\,\,\,7,6,6,4,4,3,2,2$$
$${\rm I}V.$$$$\,\,\,\,\,8,7,7,6,4,2,1,1$$
If the eigen values of $$A$$ are $$4$$ and $$8$$ then
if (i%2==0) {
if (i < n) request Ri;
if (i+2 < n) request Ri+2 ;
}
else {
if (i < n) request Rn-i;
if (i+2 < n) request Rn-i-2;
}
In which one of the following situations is a deadlock possible?$${\rm I}.$$ Shortest remaining time first scheduling may cause starvation
$${\rm II}.$$ Preemptive scheduling may cause starvation
$${\rm III}.$$ Round robin is better than $$FCFS$$ in terms of response time
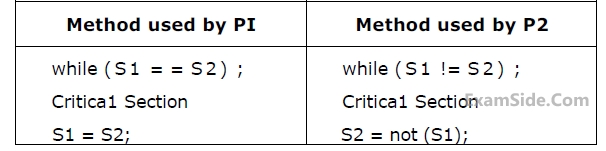
Which of the following statement describes the properties achieved?
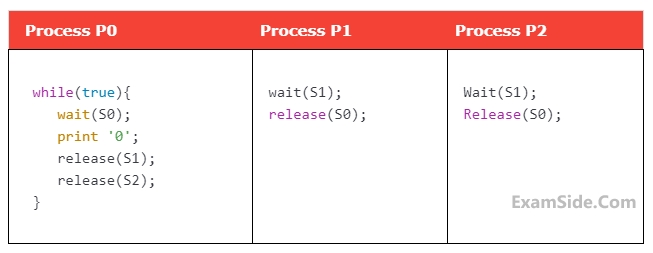
#include < stdio.h >
void f (int *p, int *q) {
p = q;
*p = 2;
}
int i = 0, j = 1;
int main ( ){
f(&i, &j);
printf ("%d %d \ n", i, j);
return 0;
}
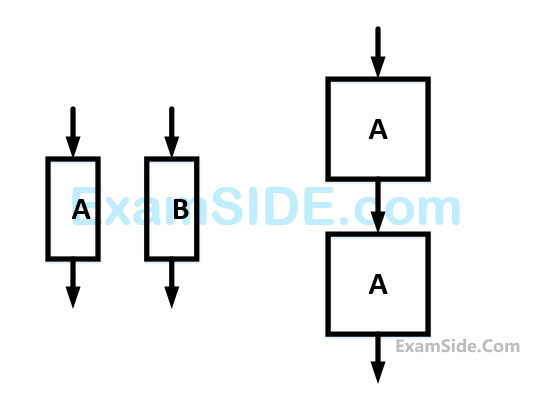
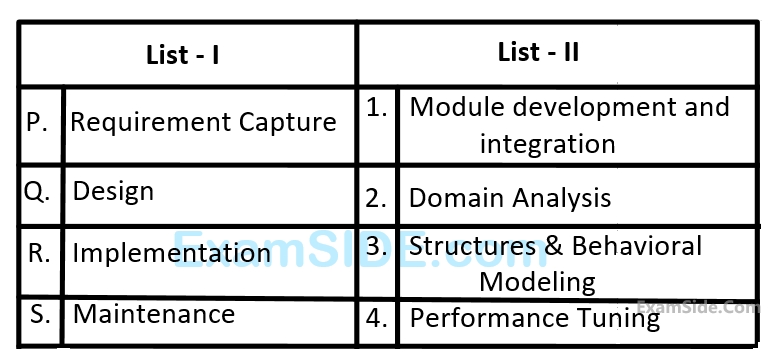
begin
if $$\left( {a = \,\, = b} \right)\,\,\left\{ {S1;\,\,exit;} \right\}$$
else if $$\left( {c = \,\, = d} \right)\,\,\left\{ {S2;} \right\}$$
else $$\left\{ {S3;\,\,exit;} \right\}$$
$$S4;$$
end
The test cases $${T_1},\,{T_2},\,{T_3}\,\,\& \,{T_4}$$ given below are expressed in terms of the properties satisfied by the values of variables $$a, b, c$$ and $$d.$$ The exact values are not given.
$${T_1}:\,a,\,b,\,c\,\& \,d$$ are all equal
$${T_2}:\,a,\,b,\,c\,\& \,d$$ are all distinct
$${T_3}:\,a = b\,\,\,\& \,\,\,\,c\,!\, = \,d$$
$${T_4}:\,a! = b\,\,\,\& \,\,\,\,c\, = \,d$$
Which of the test suites given below ensures coverage of statements $${S_1},\,{S_2},\,{S_3}\,\,\& \,{S_4}$$ ?